Accessing the Data
by Alyse Delaney, December 2022
A year after the Met announced their Open Access initiative, they launched the official Met Museum API in 2018. The API is the primary way to access the available data. Additionally, a CSV file containing every single record is available for download. The API is helpful to anyone with a moderate background in web development to engage with the data. The API has the benefit of targeting specific filters when searching the collection and thus resulting in a more targeted dataset. This is useful for developers, creators, and makers who don't necessarily need the entirety of the information. The CSV file, on the other hand, is more useful to data scientists, researchers, and students who require a complete dataset to analyze. It can be parsed using popular coding languages such as Python*. Both the API documentation and the CSV file can be found on the museum's GitHub page.
*via Celebrating Three Years of Open Access at The Met, Sofie Andersen & Spencer Kiser
How does the API work?
On the most basic level, the API provides the opportunity for a user or application to request and retrieve data from the collection. Every artwork in the collection has a unique Object ID, which can be used to find more information about the work. There are four endpoints in the Met's API. An endpoint is a URL that enables access to the information on the server.* For the Met these endpoints are: Objects, Object, Departments, and Search:
*via Endpoint vs. API, Steven Curtis
- Objects (plural) provides a list of all available object ID
- Object (singular) provides the record for the called artwork, including all of its metadata
- Departments provides a list of all department IDs and their names
- Search allows the user to find all object IDs meeting certain criteria*
*via The Metropolitan Museum of Art Collection API
Typically, the user would perform a Search to retrieve all relevant object IDs pertaining to their query. The available search fields are listed in the API documentation. For instance, if a researcher wants to find images on all paintings by Gustave Courbet in the collection, they can perform the following call:
https://collectionapi.metmuseum.org/public/collection/v1/search?hasImages=true&q=Gustave%20Courbet
This request is first targeting all records that have images (hasImages=true), then asking a question (q=) to search within those records for artists named Gustave Courbet. The result is a JSON file containing 89 object IDs. The object IDs can then be used to retrieve the artwork's full record. For instance, “Woman in the Waves” is object 436004, and can make the following call using the Object endpoint:
https://collectionapi.metmuseum.org/public/collection/v1/objects/436004
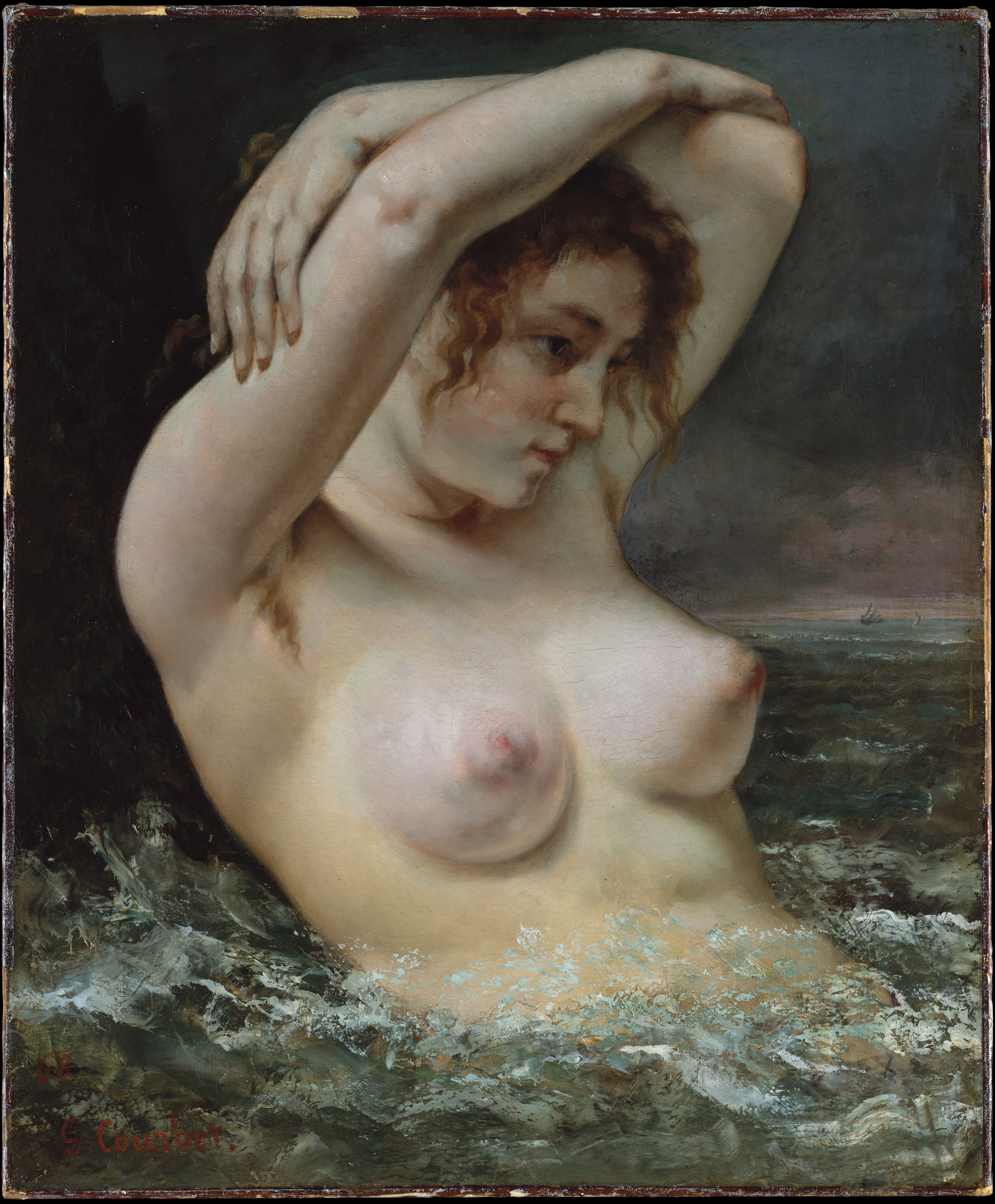
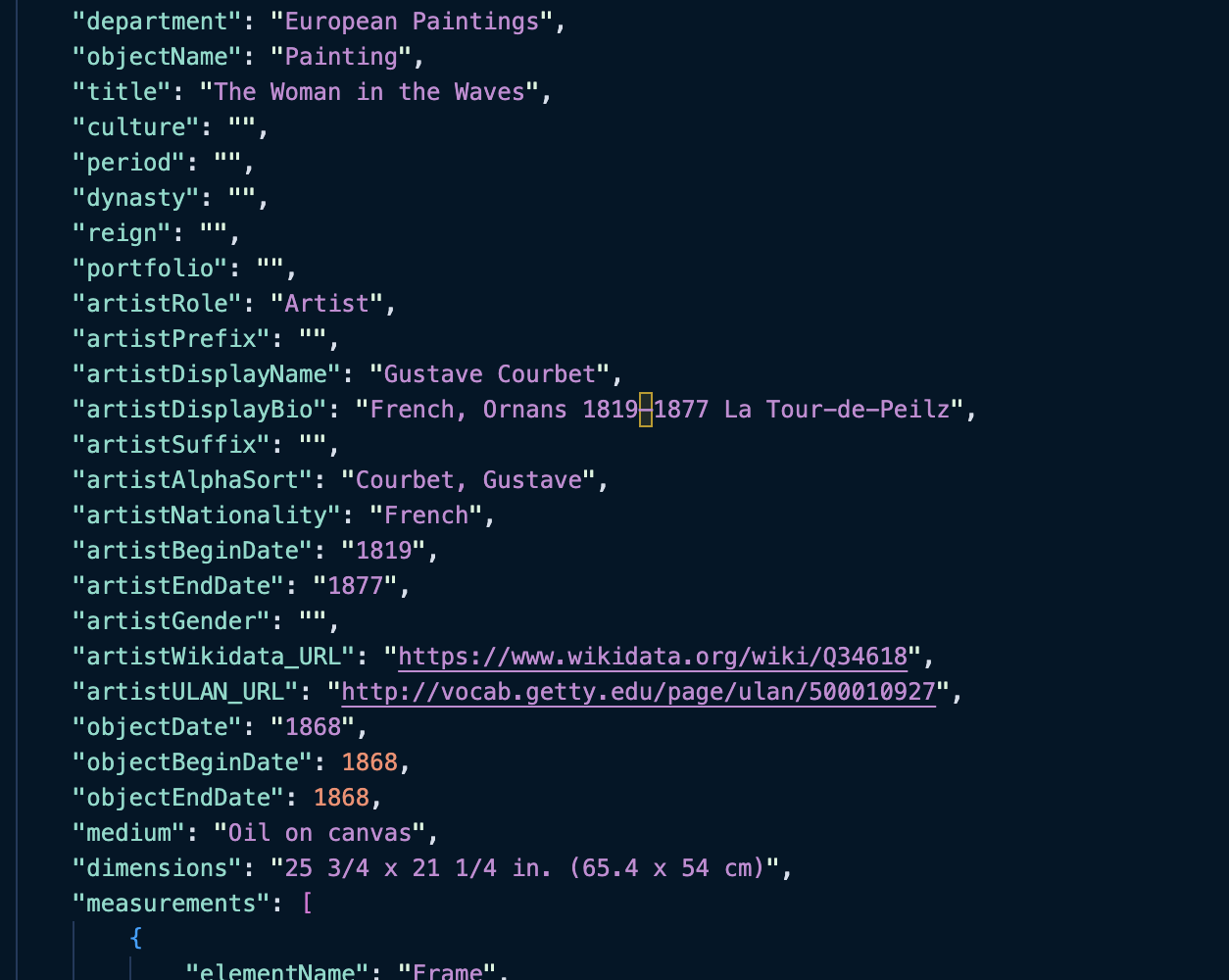
Left (Figure 1): Courbet, Gustave. Woman in the Waves. 1868. Right (Figure 2): Screenshot of the JSON file containing the painting's metadata.
As a result, we get the artwork's metadata record (Figure 2), including common tombstone fields like title, date, and medium, as well as information about the last update to the metadata, the painting's acquisition date, and links to controlled vocabulary fields such as the Getty's Union List of Artist Names, the Art & Architecture Thesaurus for tagging keywords in the artwork, and the object's Wikidata URL. As will be discussed in the next section, these fields are important for connecting the artwork to larger semantic knowledge systems. Overall, the API provides extensive access to the collection data. It does not require an API key to use, which makes it a very good learning tool for students and researchers new to APIs*
*again, via the API documentation
The Met Museum API in Action
The following mini-application uses the Met Museum API to generate a random item currently on view in the Greek and Roman Art department:
How it's Made:
The code asks the API what artworks are currently on display in the Met's Greek and Roman Art wing, then places the information into HTML code using JavaScript.
First, the following code snippet calls to the API. It asks the API to retrieve the Object IDs for works of art that are 1) from Greek and Roman Art (Department 13), 2) are currently on view (isOnView=True), and 3) have images (hasImages=true).
fetch('https://collectionapi.metmuseum.org/public/collection/v1/search?departmentId=13&isOnView=true&hasImages=true&q=*')
.then((response) => response.json())
After that, it generates a random number to select from the array of objects provided by the API to randomly select one of the object IDs:
.then((objects) => {
const randomSelect = Math.floor(Math.random()*objects.total)
const randomObject = objects.objectIDs[randomSelect]
The random object ID is then placed into another call to the API:
let objectCall = `https://collectionapi.metmuseum.org/public/collection/v1/objects/${randomObject}`
fetch(objectCall)
.then((response) => response.json())
The resulting information is then inserted into the related HTML elements:
.then((data) => {
document.getElementById("random-artwork-image").src=data.primaryImageSmall
document.getElementById("artwork-title").innerText=data.title
document.getElementById("artwork-period").innerText=data.period
document.getElementById("artwork-objectdate").innerText=data.objectDate
document.getElementById("artwork-medium").innerText=data.medium
document.getElementById("artwork-culture").innerText=data.culture
document.getElementById("artwork-gallery").innerText=data.GalleryNumber
document.getElementById("artwork-link").href=data.objectURL
The entire script is placed in a function called "randomArt()", which is then used to create the Randomize button.
← Previous: Introduction